Microchip Filter Design
Implementing FIR and IIR Digital Filters. DS00852A-page 2 2002 Microchip Technology Inc. TABLE 1: FIR FILTER. The user must design the filter.
X is a constant (I should have used k) and represents the number of samples in your filter. Using the values in your example, your output would be lastreadingoutput 012816 1613030 3014044 4412855 It will take a while for the filter output to approximate your reading, but I hope you get the idea. The one issue that you either have to use floating point math or maintain the remainder from each filter operation to use in the following operation (if you are using integer math). Forget what I said earlier about not using floating point. If you are using C and are not in a tight timing requirement, use floating point. It is much easier than dealing with integer math in this particular algorithm.
Hope this helps. The subject of digital filter design is too extensive to be covered completely by any of the specific posts on this board. The most you will find are rules of thumb that were used in particular projects which may or may not parallel what you are doing. It would be more productive if you defined not only the parameters of the filter (cutoff frequency, shape, etc.) but also just how serious you are about the performance. In general, digital filters are implemented in two broad ways. In the first, the output is the weighted sum of the last N inputs.
Each input (the past N-1 and the current one) is multiplied by a different 'coefficient' and the products are added together to get the current output. These are the 'Finite Impulse Response' or FIR filters.
The coeficients determine the filter response (shape and cutoff frequency). This is where the math and theory come in. The IIR (Infinite Impulse Response) filters constitute the second type. The output of these is the weighted sum of the last N inputs and the last M outputs.
This type can produce more complicated stop and pass characteristics with fewer stages (coefficients), at the expense of potentially unstable behavior (oscillation and overshoot, e.g.). As with the FIR, calculation of the coefficients is math intensive. If you don't care about precision (the performance mentioned above), use a simple averaging algorithm. To use jargon: make an FIR filter with all coefficients equal to 1/N.
This is the classic 'boxcar integrator'. The response is sin(f)/f, which has a bumpy shape and works best if you just want DC.
If you need a fancier design, make a simple exponentially shaped filter using (formula edited) output = (1 -a). input + a. previousoutput, 0. The problem that I have had with using straight integer math like this is that you can never reach a steady state input because of loss of precision. The longer your filter period, the further away you are from steady state. Even rounding won't get you there.
If you use a period of eight and change from 0 to an ADC reading of 483, you would reach a filtered reading of 480 after 36 iterations, and would never exceed 480. This is because you are losing the remainder of your integer operation. I have found that you need to keep the LOB (low order byte) of the operation (even if you don't output it) and use it when the next output is calculated. For a sample weight of 255, the difference is even more dramatic. The math is still integer and still very fast, but the extra byte has to be handled.
Of course someone probably knows another trick that I don't. Well this is a little long but difference equations translate int C very well: Let $ = integral (had to use some character) Let Xn = X at sample time n Let Xn-1 = X at sample time n-1 A first order filter in the laplace domain is Y/X = 1/(tau.s + 1 ) where: x=input y=output s=laplace operator tau = time constant in sec/rad w = 1/tau = break frequency in rad/sec (Note 2.pi rad = 1cycle) You can arrange the equation above like this Y(tau.s+1) = X. Multiply denominators tau.Y.s + Y = X.
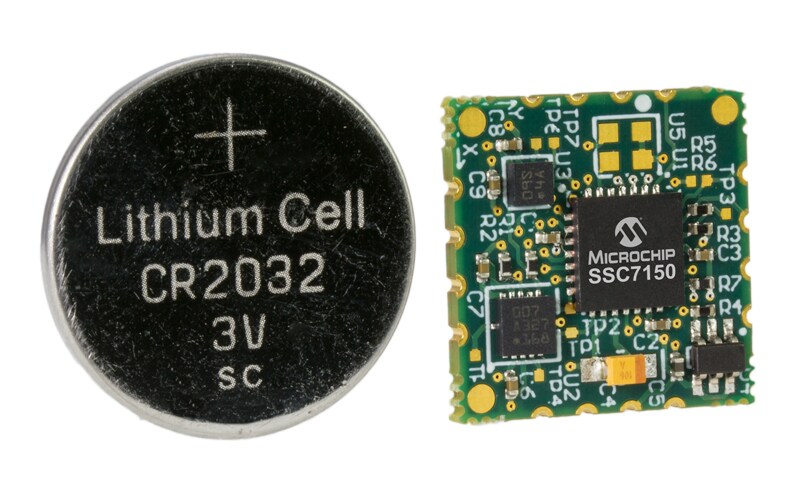
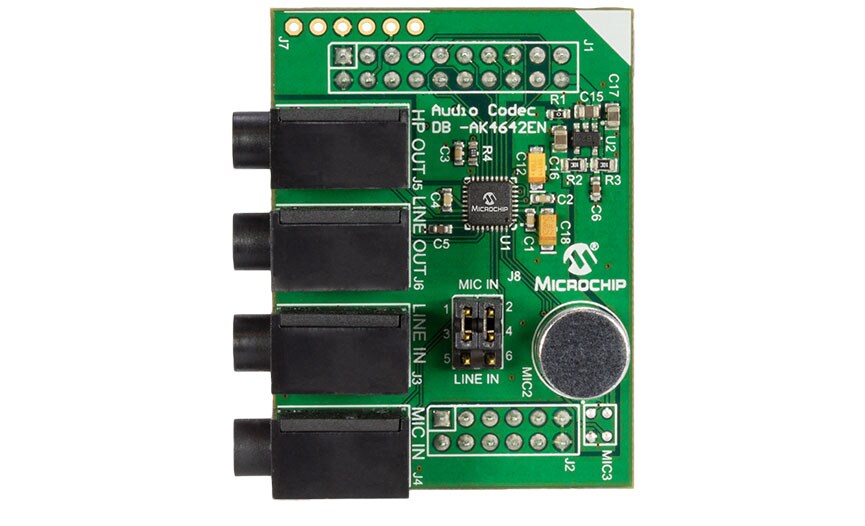
Expand equation tau.Y + Y/s = X/s. Divide by the laplace operator s Now our knowledge of lapace operators is Z/s = $Z.
Passive Filter Design
The integral of Z Substituting into the equation we get tau.Y + $Y = $X. Tranfering from lapace domain to time domain If this is true continuously, we can say for small delta time steps (n) it is also true tau.Yn + $Yn = $Xn. Discretized version (A) There are many types of integration routines but for small delta time steps let's use rectangular integration $Zn = $Zn-1 + dt.Zn substituting into (A) we get tau.Yn + $Yn-1 +dt.Yn = $Xn-1 + dt.Xn. Integral Substitution (B) Now if Equation A is true for n, it must also be true for n-1 tau.Yn-1 + $Yn-1 = $Xn-1. Discretized to n-1 (C) Now the fun part, the difference equation (B - C).